Read Text File and Display in Html Using Angular 4
You can brandish data by binding controls in an HTML template to properties of an Angular component.
In this folio, you'll create a component with a listing of heroes. You'll display the listing of hero names and conditionally show a message beneath the list.
The last UI looks like this:
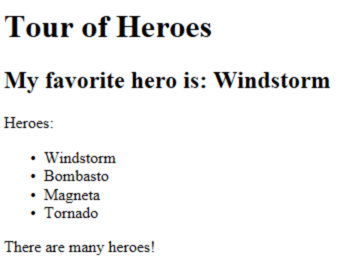
Contents
- Showing component properties with interpolation.
- Showing an assortment holding with NgFor.
- Conditional display with NgIf.
The
Showing component properties with interpolation
The easiest way to display a component property is to bind the property proper name through interpolation. With interpolation, yous put the property proper name in the view template, enclosed in double curly braces: {{myHero}}
.
Follow the setup instructions for creating a new project named
Then modify the
When yous're washed, it should look like this:
src/app/app.component.ts
You lot added two properties to the formerly empty component: title
and myHero
.
The revised template displays the two component properties using double curly brace interpolation:
The template is a multi-line string within ECMAScript 2015 backticks (`
). The backtick (`
)—which is non the aforementioned character as a unmarried quote ('
)—allows you to etch a string over several lines, which makes the HTML more readable.
Angular automatically pulls the value of the title
and myHero
properties from the component and inserts those values into the browser. Angular updates the display when these properties change.
More precisely, the redisplay occurs later on some kind of asynchronous upshot related to the view, such equally a keystroke, a timer completion, or a response to an HTTP asking.
Notice that yous don't call new to create an instance of the AppComponent
class. Angular is creating an instance for you. How?
The CSS selector
in the @Component
decorator specifies an element named <my-app>
. That chemical element is a placeholder in the body of your alphabetize.html
file:
src/index.html (body)
When you bootstrap with the AppComponent
course (in <my-app>
in the index.html
, finds it, instantiates an case of AppComponent
, and renders information technology within the <my-app>
tag.
Now run the app. Information technology should display the title and hero proper name:
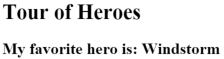
The next few sections review some of the coding choices in the app.
Template inline or template file?
You tin can shop your component'south template in one of two places. You can ascertain it inline using the template
holding, or you tin can define the template in a split HTML file and link to information technology in the component metadata using the @Component
decorator's templateUrl
property.
The choice between inline and separate HTML is a matter of taste, circumstances, and organization policy. Hither the app uses inline HTML because the template is modest and the demo is simpler without the boosted HTML file.
In either style, the template information bindings have the same access to the component's properties.
Constructor or variable initialization?
Although this case uses variable assignment to initialize the components, you can instead declare and initialize the backdrop using a constructor:
src/app/app-ctor.component.ts (class)
This app uses more terse "variable assignment" fashion simply for brevity.
Showing an array property with *ngFor
To display a list of heroes, begin by adding an array of hero names to the component and redefine myHero
to exist the beginning name in the array.
src/app/app.component.ts (class)
At present use the Angular ngFor
directive in the template to display each item in the heroes
list.
src/app/app.component.ts (template)
This UI uses the HTML unordered list with <ul>
and <li>
tags. The *ngFor
in the <li>
chemical element is the Angular "repeater" directive. It marks that <li>
element (and its children) as the "repeater template":
Don't forget the leading asterisk (*) in *ngFor
. It is an essential part of the syntax. For more information, run across the Template Syntax page.
Notice the hero
in the ngFor
double-quoted instruction; it is an example of a template input variable. Read more than about template input variables in the microsyntax department of the Template Syntax page.
Angular duplicates the <li>
for each detail in the list, setting the hero
variable to the item (the hero) in the current iteration. Angular uses that variable every bit the context for the interpolation in the double curly braces.
In this case, ngFor
is displaying an array, but ngFor
tin repeat items for any iterable object.
At present the heroes appear in an unordered listing.
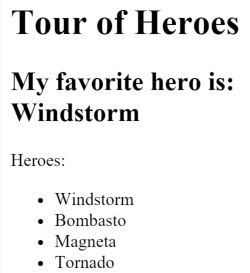
Creating a class for the data
The app'south code defines the data direct inside the component, which isn't all-time practice. In a elementary demo, however, it's fine.
At the moment, the binding is to an array of strings. In real applications, most bindings are to more specialized objects.
To convert this binding to employ specialized objects, turn the array of hero names into an array of Hero
objects. For that y'all'll need a Hero
class.
Create a new file in the app
folder called
src/app/hero.ts (extract)
Y'all've defined a class with a constructor and two properties: id
and name
.
It might non look like the class has backdrop, but information technology does. The declaration of the constructor parameters takes advantage of a TypeScript shortcut.
Consider the first parameter:
src/app/hero.ts (id)
That brief syntax does a lot:
- Declares a constructor parameter and its type.
- Declares a public holding of the same name.
- Initializes that property with the corresponding statement when creating an case of the grade.
Using the Hero course
After importing the Hero
course, the AppComponent.heroes
property can return a typed array of Hero
objects:
src/app/app.component.ts (heroes)
Next, update the template. At the moment information technology displays the hero's id
and name
. Set that to display only the hero's name
property.
src/app/app.component.ts (template)
The brandish looks the same, but the code is clearer.
Conditional brandish with NgIf
Sometimes an app needs to brandish a view or a portion of a view only under specific circumstances.
Let'due south alter the instance to brandish a message if there are more than three heroes.
The Athwart ngIf
directive inserts or removes an chemical element based on a truthy/falsy condition. To run across it in action, add together the following paragraph at the bottom of the template:
src/app/app.component.ts (message)
Don't forget the leading asterisk (*) in *ngIf
. It is an essential part of the syntax. Read more most ngIf
and *
in the ngIf section of the Template Syntax page.
The template expression inside the double quotes, *ngIf="heros.length > iii"
, looks and behaves much like TypeScript. When the component's list of heroes has more than three items, Athwart adds the paragraph to the DOM and the message appears. If in that location are 3 or fewer items, Angular omits the paragraph, and then no message appears. For more data, see the template expressions section of the Template Syntax folio.
Angular isn't showing and hiding the bulletin. It is adding and removing the paragraph element from the DOM. That improves performance, especially in larger projects when conditionally including or excluding big chunks of HTML with many information bindings.
Try it out. Considering the array has four items, the message should appear. Go dorsum into
Summary
Now you know how to utilize:
- Interpolation with double curly braces to brandish a component property.
- ngFor to display an array of items.
- A TypeScript class to shape the model data for your component and display backdrop of that model.
- ngIf to conditionally brandish a chunk of HTML based on a boolean expression.
Here'south the terminal code:
Source: https://v2.angular.io/docs/ts/latest/guide/displaying-data.html
0 Response to "Read Text File and Display in Html Using Angular 4"
Post a Comment